Vue convert image to Base64: Base64 is a method of encoding binary data into text format for easier storage, transmission, and decoding. In Vue.js, to convert an image to Base64, you need to first retrieve the image data. This can be done by using the FileReader API. Then, use the btoa function to encode the image data into Base64 format. The resulting text string can be used as a data URL, embedded in HTML, or sent to a server for storage. It’s important to note that Base64 encoding increases the size of the original data by about 33%.
How to convert Vue Image to Base64 string
This Below Example explain you to implementation of Vue convert image to Base64 string
- An HTML
<div>
with an id ofapp
is created, which serves as the root element for the Vue app. - Within the
<div>
, there’s an input field of type “file” with a@change
event listener. - Below the input field, there’s a
<textarea>
element that displays the Base64 string and is bound to thebase64textString
data property usingv-model
. - In the script tag, a new Vue instance is created with the following properties:
el
property is set to the#app
element, which serves as the root element for the Vue app.data
property returns an object containing thebase64textString
property, which is initially set to an empty string.methods
property contains a single method calledconvertToBase64
that’s invoked when the file input’s value changes.
- In the
convertToBase64
method, the following steps are performed:- The first file in the file list is extracted using
event.target.files[0]
. - A new
FileReader
object is created. - The
readAsDataURL
method is called on theFileReader
object, passing the file as an argument. - The
onload
event listener is added to theFileReader
object, which sets the value ofbase64textString
to the result of thereadAsDataURL
method. - An
onerror
event listener is also added to log any errors that might occur during the reading of the file.
- The first file in the file list is extracted using
Vue Convert Image to Base64 Example
<div id="app">
<input type="file" @change="convertToBase64" accept="image/*" />
<br />
<div v-if="showImage">
<img :src="base64textString" :alt="imageName" />
</div>
<br />
<textarea v-model="base64textString" rows="5"></textarea>
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
base64textString: '',
imageName: '',
showImage: false
};
},
methods: {
convertToBase64(event) {
const file = event.target.files[0];
this.imageName = file.name;
const reader = new FileReader();
reader.readAsDataURL(file);
reader.onload = () => {
this.base64textString = reader.result;
this.showImage = true;
};
reader.onerror = (error) => {
console.log('Error: ', error);
};
}
}
});
</script>
Output of Vue Convert Image to Base64
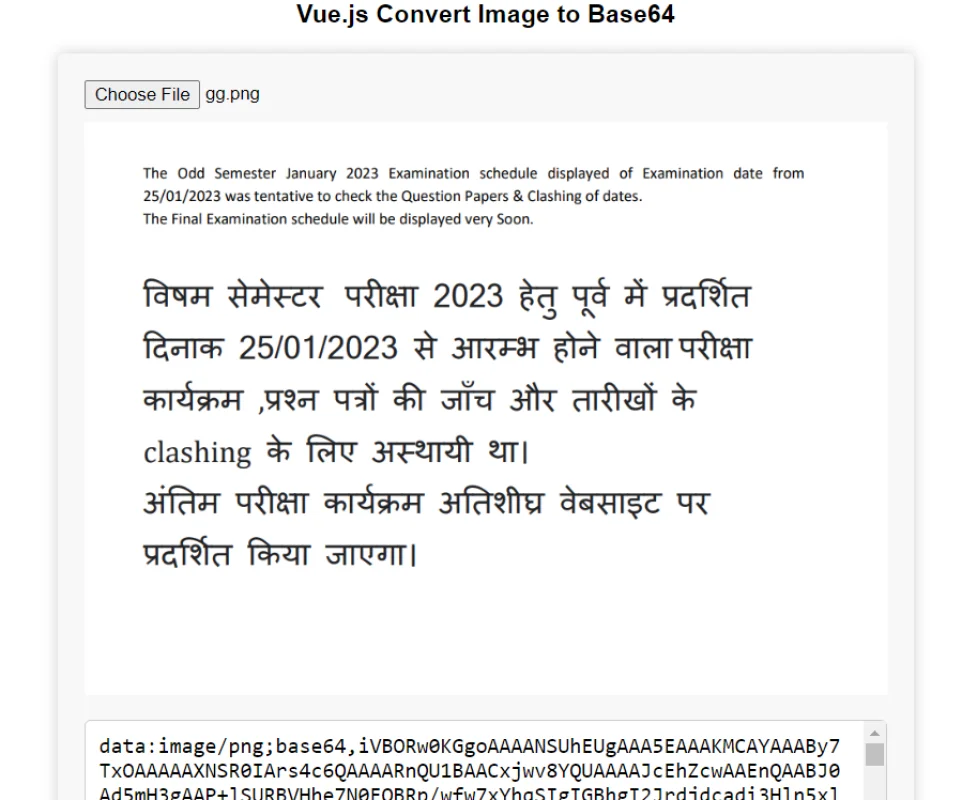